Many websites publish blog posts written by authors that do not have accounts configured on the particular WordPress website. By default the post author is going to be the name of the person that created the post entry, not the actual writer. You can configure your blog to hide the author’s name on all posts, but it would be even better if you could list the name of the real post author instead.
This code snipped will allow you to do just that. It adds a field to your post sidebar when you are editing. If you leave the guest author field empty, WordPress will display the WordPress user name in the post author field. If you enter a name, that name will be displayed instead.
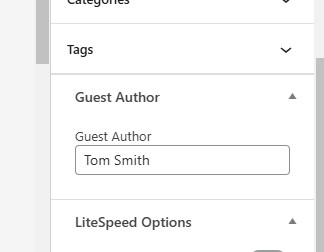
// Add the meta box to the post edit screen
function add_guest_author_meta_box() {
add_meta_box(
'guest_author_meta_box', // ID
'Guest Author', // Title
'display_guest_author_meta_box', // Callback function
'post', // Post type
'side', // Context (side, advanced, normal)
'high' // Priority (low, default, high)
);
}
add_action('add_meta_boxes', 'add_guest_author_meta_box');
// Display the meta box
function display_guest_author_meta_box($post) {
// Retrieve the current guest author value based on the post ID
$guest_author = get_post_meta($post->ID, '_guest_author', true);
?>
<label for="guest_author">Guest Author</label>
<input type="text" name="guest_author" id="guest_author" value="<?php echo esc_attr($guest_author); ?>" class="widefat" />
<?php
}
// Save the meta box data when the post is saved
function save_guest_author_meta_box_data($post_id) {
// Check if our nonce is set.
if (!isset($_POST['guest_author_nonce'])) {
return $post_id;
}
$nonce = $_POST['guest_author_nonce'];
// Verify that the nonce is valid.
if (!wp_verify_nonce($nonce, 'save_guest_author_meta_box_data')) {
return $post_id;
}
// Check if this is an autosave.
if (defined('DOING_AUTOSAVE') && DOING_AUTOSAVE) {
return $post_id;
}
// Check the user's permissions.
if (isset($_POST['post_type']) && 'post' == $_POST['post_type']) {
if (!current_user_can('edit_post', $post_id)) {
return $post_id;
}
}
// Sanitize user input.
$guest_author = sanitize_text_field($_POST['guest_author']);
// Update the meta field in the database.
update_post_meta($post_id, '_guest_author', $guest_author);
}
add_action('save_post', 'save_guest_author_meta_box_data');
// Add a nonce field to the meta box form for security
function add_guest_author_meta_box_nonce() {
wp_nonce_field('save_guest_author_meta_box_data', 'guest_author_nonce');
}
add_action('edit_form_after_title', 'add_guest_author_meta_box_nonce');
// Replace author name with "Guest" in blog archive listings
function replace_author_name_with_guest($display_name) {
$id = get_the_ID();
$guest_author = get_post_meta($id, '_guest_author', true);
// Check if it's an archive page
if ($guest_author != '') {
$display_name = $guest_author;
}
return $display_name;
}
add_filter('the_author', 'replace_author_name_with_guest');
Happy Blogging!